Guidelines:
COM+ Component Design
This material is provided courtesy of Applied Information
Sciences, Inc.
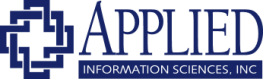
Topics
References 
Summary 
These guidelines provide information on considerations that the
software architect and designer should include in the design of components for Microsoft
Windows DNA®-based applications. These guidelines are not intended to provide
complete coverage of all topics associated with the design of components using
Microsoft’s Component Object Model (COM) technology. The focus of these
guidelines is on three key design mechanisms that are available in the Microsoft
Windows® 2000 version of the COM, referred to as COM+. These design mechanisms
are transactions, queued components, and loosely coupled events (LCE).
COM+ components are physical, nearly independent parts of a
Windows DNA-based application. COM+ components contain one or more application
classes that expose methods through interfaces. Component interfaces are
considered to be “contracts” between the component and clients of the
component. Existing methods of published interfaces should not be modified, but
new methods may be added to the interface.
The classes in the COM+ components can also implement operations
for pre-defined methods that are provided by components included in the COM+
services infrastructure. COM+ components are typically generated as either a
dynamic link library (DLL) or as an executable application (EXE).
With the release of the Microsoft Windows 2000 operating system,
Microsoft has integrated the transaction and process control services of the
Microsoft Transaction Server® (MTS) with the component management and
infrastructure services provided in the original COM technology. Together they
define they specification for the next generation of components, referred to as
COM+. In addition to merging MTS and COM together, Microsoft also integrated new
services into the COM+ specification. Some of the major new services provided
are: queued components, loosely coupled events, object pooling, role based
security, and so on. Refer to the Microsoft Developer Network (MSDN) Web site msdn.microsoft.com
for a complete description of the latest COM+ services.
In the Artifact: Design Model,
the following modeling elements are utilized in designing COM+ components for
Windows DNA-based applications:
-
Design Classes – Model the classes contained in the COM+
components.
-
Design Subsystems – Model the logical grouping (packaging)
of classes into COM+ Components.
-
Interfaces – Model the component interfaces that contain
the exposed operations of the component.
-
Design Packages – Used to model COM+ Applications.
See the following topic areas for more information on each of
these design elements: Guidelines: Design Class, Guidelines:
Design Subsystem, Guidelines: Interfaces, and Guidelines:
Design Package. See the Tool
Mentors: Managing the Design Model Using Rational Rose, Managing
Classes Using Rational Rose, and Managing
Subsystems Using Rational Rose for general guidance on how to create these
design elements in Rational Rose.
There are many useful pre-defined stereotypes for modeling COM+
classes. Some of the most important are the Unified Modeling Language (UML) Web
Application Extensions (WAE). These stereotypes can be used to model important
design elements, such as Active Server Pages (ASPs) for web-hosted Windows
DNA-based applications. See the Rational Rose help topic “Rose Web Modeler”
for more information on the WAE class stereotypes.
In addition to the WAE stereotypes, Rational Rose also has
Windows DNA specific class and component stereotypes that are useful in modeling
COM+ classes and components. See the Rational Rose Help topic sections on “Rose Visual Basic”
and “Rose Visual C++” for detailed information on using the Windows DNA
specific stereotypes and detailed information on modeling Windows DNA-based
applications implemented using Microsoft Visual Basic® and Microsoft Visual C++®
technologies.
As described in Concepts:
Design and Implementation Mechanisms, design mechanisms represent a
common pattern or solution architecture for a specific problem domain. This
section will explain some of the considerations for using three specific types
of COM+ design mechanisms that the software architect and designer may use when designing
COM+ classes and components during the Activity:
Class Design and Activity: Subsystem
Design in the Workflow Detail:
Design Components. The three types of services that are discussed in these
guidelines are transactions, queued components, and events.
Description
In general terms a transaction is a unit of processing
consisting of one or more application components initiated by a single request.
In COM+ terms, a transaction is typically a unit of work in which all of the
objects participating must complete work as a single unit to update a specific
data store.
Transactional components are those components whose objects
communicate with a data store. The actions performed by the component are things
that must be committed at a single time. In other words, if one piece of
functionality fails, then the entire transaction fails. This prevents incomplete
transactions. For example, if while attempting to transfer $100.00 from your
savings account to your checking account, the transaction fails after debiting
$100.00 from your savings account but before crediting your checking account,
then it is desirable to have the entire transaction undone. If not then you are
out $100.00. Your savings account was debited, but no credit was performed.
To help decide which classes and components are transactional,
the designer should consider using sequence diagrams to depict all of the object
interactions that occur in the scope of the transaction. The designer may use
proxy classes to represent components in the sequence diagram. The figure below
shows an example of a sequence diagram that models a COM+ transaction.
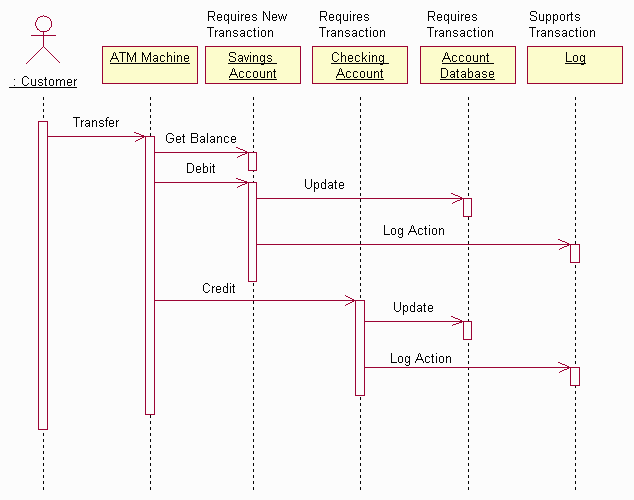
Sequence Diagram for a Sample Transaction
Design Considerations
When designing components, a designer should evaluate whether a
given component is transactional or non-transactional.
Transactional components are those components that participate
in data store transactions. The components determined to be transactional
require more design thought than the non-transactional components. COM+ provides
support for designating components as one of the following:
If a component modifies information in a data store where data
integrity must be guaranteed, the component should be designed as requiring
transactions. If a component provides operations that a transactional component
may use, but the component does not directly modify the data store, then the
component should be designed as supporting transactions
Visual Basic components participating in transactions must
include implementation for the IObjectContext COM+ interface. When packaging
classes, those classes that participate in the same logic unit of work, or in a
single transaction, should be packaged into a single component. Those classes in
the component that implement the transaction must be public classes.
Description
Queued components are components that participate in an
asynchronous environment. The desired actions are queued until the system is
capable of performing desired business functionality. Queued components are also
utilized in disconnected environments. For example, functionality can be queued
on a laptop in the field, the information and processing based upon actions
while the laptop was in the field are performed when the laptop is reconnected
to a network. This means that the laptop is configured with a queued component
recorder and the network business application is configured with a queued
component listener. When the listener makes contact with the laptop, the actions
queued on the laptop are processed on the business server. The figure below
depicts the support architecture for queued components.
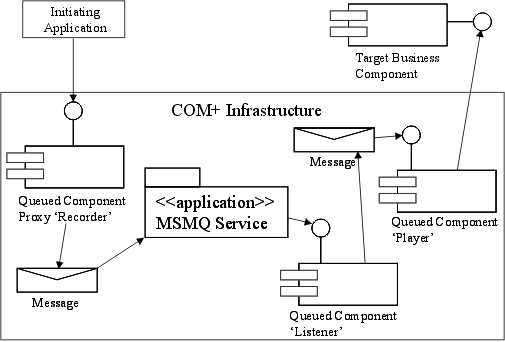
Queued Component Architecture
Design Considerations
The basic design consideration for using queued components is
whether the application must perform all operations while the
calling routine is “connected” (synchronously) or whether there are
functions of the application that can be done in an “off-line” or deferred
manner.
Some specific situations that the designer should consider using
queued components for are as follows:
-
Application processing load management – Queued components
provide a means of spreading out the processing load of an application by
allowing processing requests that do not require immediate action to be
queued up for dispatching during non-peak load hours.
-
Managing mobile users access to applications and data –
Queued components can be used to allow mobile, disconnected users to enter
data into the application front end on their lap-top then have the
information queued up to processing later, when they are able to connect to
the back-end database support systems.
-
Managing application failures – Queued components can be
employed as a failure support function to handle situations in which an
application that normally processes user interactions synchronously,
experiences failures on the back end systems and cannot process the user
requests. Queued components could be used to provide limited front-end
processing and store requests for later execution once the application
back-end systems are made operational.
The designer should keep in mind that when using queued
components that the message queuing services do not provide automatic
notification that a queued processing request has been dispatched and has
completed processing. The designer must consider whether or not to implement a
notification scheme for queued processing requests.
The designer also needs to keep in mind that parameters to
methods in queued components must be passed by value, ByVal. The interface
cannot be queued if it contains ByRef parameters.
Description
COM+ events utilize a publish and subscribe model. Publishers
are components that “publish” a set of interface operations that are used to
trigger the events. The interface operations for the events are stored in the
COM+ catalog. Subscribers are components that provide implementations of the
operations in the “published” event interface. Subscriber components must
also register their “subscriptions” to the events in the COM+ catalog.
Events are fired when a component invokes one of the operations in the event
interface. There can be multiple subscribers to a particular event. The COM+
events infrastructure provides services for filtering the receipt of the events by the subscriber
components. The figure below depicts the general COM+ events architecture.
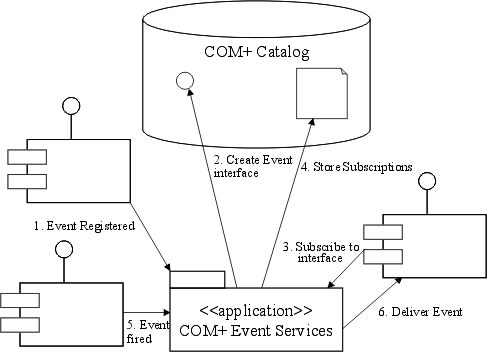
COM+ Event Services Architecture
Design Considerations
When designing events, you must consider whether a component can
be accessed directly through a component’s interface and through COM+ event
service, or if the component can be accessed only through the COM+ event
service.
In Visual Basic, an interface is created for each public class
in a component. If you create a public class for a component then register that
component to subscribe to an event in COM+ services, there are actually two
interfaces for the same implementation of the component. The component can be
accessed directly through the interface created when the component was compiled
in Visual Basic. The component can also be accessed through COM+ services when
the interface to the component subscribed is fired.
If the designer wishes to create a single interface for the
component, one that is defined by subscribing to the interface in COM+ services,
then it is necessary to define a private class (if that is done, no interface is
created when Visual Basic compiles the component). The component itself will
contain an implements statement for the interface published in COM+ services.
Copyright
© 1987 - 2001 Rational Software Corporation
|